Working with JSON in C# 9 and .NET Core 5
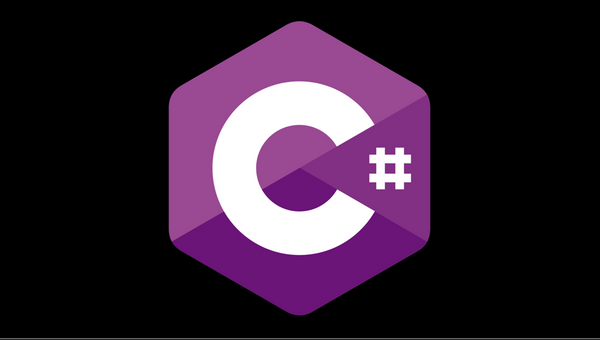
System.Text.Json
If you've ever worked with JSON objects in C#, you've probably heard of the NewtonSoft.Json
library. While it's still one of the most popular libraries for serializing and deserializing JavaScript objects, Microsoft's new System.Text.Json
library brings native support from the language and several performance improvements under the hood.
To start using these new tools simply include these in your .NET projects:
using System.Text.Json;
using System.Text.Json.Serialization;
A quick example of serializing a Person
class with these new tools:
using System.Text.Json;
using System.Text.Json.Serialization;
class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
}
string Serialize(Person person)
{
return JsonSerializer.ToString<Person>(person);
}
If you're looking for more samples, check out the documentation from Microsoft.
Records
I've been working with C# more and more since joining Microsoft in 2019. Most of the applications I build are backend applications that serve JSON to customers. While Records aren't explicitly related to JSON, I have so many C# classes that could easily be replaced with Records.
We have tons of classes that look something like this:
public class Person
{
public string LastName { get; set; }
public string FirstName { get; set; }
public Person(string first, string last) {
FirstName = first;
LastName = last;
}
}
Well, with C# 9's new record
type, we can do something like this:
public record Person (string FirstName, string LastName);
These are called positional records and achieve the same thing with fewer lines of code. If your application has a lot of objects like this to interact with, you can clean up your code base by creating these very brief statements.
You can still do many operations that you would do on classes, but an important note here is that these are meant to be immutable objects. As soon as the object has been created, it cannot be changed. This feature allows developers to guarantee objects aren't being modified after creation. If you wanted to achieve this in previous versions of C#, you would've had to write the additional code yourself.
if you're looking for more samples, check out the documentation from Microsoft.